作为你所说的“轻微边框”,实际上我们可以通过一些小技巧轻松实现。因为,如果你仔细观察,每个单元格的顶部比深色交替行略微浅一些,底部是暗灰色,你可以创建NSTableView子类,然后重写- (void)drawRow:(NSInteger)row clipRect:(NSRect)clipRect
方法:
- (void)drawRow:(NSInteger)row clipRect:(NSRect)clipRect
{
NSRect cellBounds = [self rectOfRow:row];
NSColor *color = (row % 2) ? [NSColor colorWithCalibratedWhite:0.975 alpha:1.000] : [NSColor colorWithCalibratedRed:0.932 green:0.946 blue:0.960 alpha:1.000];
[color setFill];
NSRectFill(cellBounds);
[[NSColor colorWithCalibratedWhite:0.912 alpha:1.000] set];
CGContextRef currentContext = [[NSGraphicsContext currentContext]graphicsPort];
CGContextSetLineWidth(currentContext,1.0f);
CGContextMoveToPoint(currentContext,0.0f, NSMaxY(cellBounds));
CGContextAddLineToPoint(currentContext,NSMaxX(cellBounds), NSMaxY(cellBounds));
CGContextStrokePath(currentContext);
[[NSColor colorWithCalibratedRed:0.961 green:0.970 blue:0.985 alpha:1.000] set];
CGContextSetLineWidth(currentContext,1.0f);
CGContextMoveToPoint(currentContext,0.0f,1.0f);
CGContextAddLineToPoint(currentContext,NSMaxX(self.bounds), 1.0f);
CGContextStrokePath(currentContext);
[super drawRow:row clipRect:clipRect];
}
When displayed in a tableview, it will look like this:
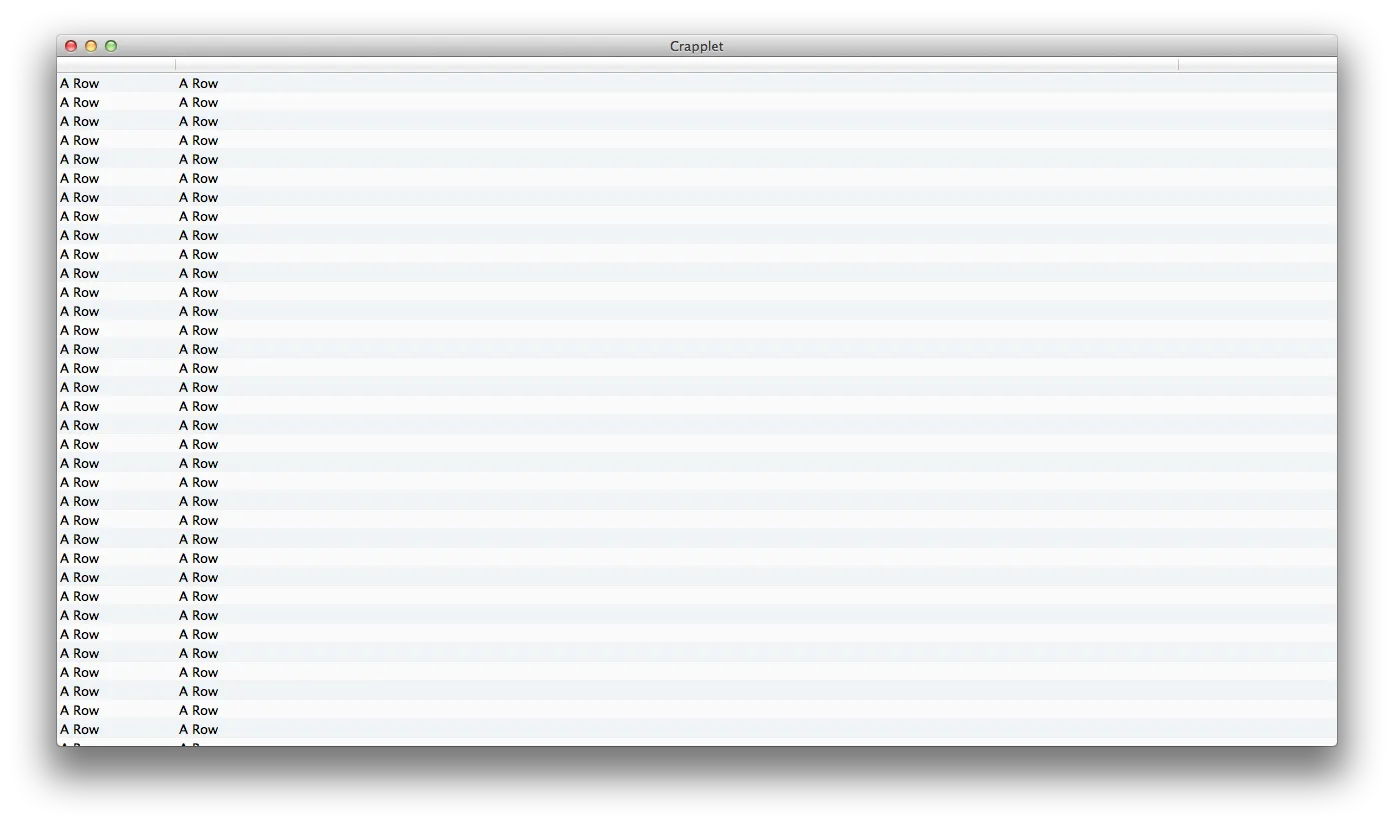
However, how should we handle the top and bottom of the tableview? They will either be an unattractive white or the default alternating row color. As Apple revealed in a talk titled "
View Based NSTableView, Basic To Advanced", you can override
-(void)drawBackgroundInClipRect:(NSRect)clipRect
and use some calculations to draw the tableview background as extra rows. A quick implementation would look something like this:
-(void)drawBackgroundInClipRect:(NSRect)clipRect
{
[super drawBackgroundInClipRect:clipRect];
CGFloat yStart = 0;
NSInteger rowIndex = -1;
if (clipRect.origin.y < 0) {
while (yStart > NSMinY(clipRect)) {
CGFloat yRowTop = yStart - self.rowHeight;
NSRect rowFrame = NSMakeRect(0, yRowTop, clipRect.size.width, self.rowHeight);
NSUInteger colorIndex = rowIndex % self.colors.count;
NSColor *color = [self.colors objectAtIndex:colorIndex];
[color set];
NSRectFill(rowFrame);
[[NSColor colorWithCalibratedWhite:0.912 alpha:1.000] set];
CGContextRef currentContext = [[NSGraphicsContext currentContext]graphicsPort];
CGContextSetLineWidth(currentContext,1.0f);
CGContextMoveToPoint(currentContext,0.0f, yRowTop + self.rowHeight - 1);
CGContextAddLineToPoint(currentContext,NSMaxX(clipRect), yRowTop + self.rowHeight - 1);
CGContextStrokePath(currentContext);
[[NSColor colorWithCalibratedRed:0.961 green:0.970 blue:0.985 alpha:1.000] set];
CGContextSetLineWidth(currentContext,1.0f);
CGContextMoveToPoint(currentContext,0.0f,yRowTop);
CGContextAddLineToPoint(currentContext,NSMaxX(clipRect), yRowTop);
CGContextStrokePath(currentContext);
yStart -= self.rowHeight;
rowIndex--;
}
}
}
但是,这样会使表格视图底部保持相同的丑陋白色!因此,我们还必须覆盖-(void)drawGridInClipRect:(NSRect)clipRect
。另一个快速实现如下:
-(void)drawGridInClipRect:(NSRect)clipRect {
[super drawGridInClipRect:clipRect];
NSUInteger numberOfRows = self.numberOfRows;
CGFloat yStart = 0;
if (numberOfRows > 0) {
yStart = NSMaxY([self rectOfRow:numberOfRows - 1]);
}
NSInteger rowIndex = numberOfRows + 1;
while (yStart < NSMaxY(clipRect)) {
CGFloat yRowTop = yStart - self.rowHeight;
NSRect rowFrame = NSMakeRect(0, yRowTop, clipRect.size.width, self.rowHeight);
NSUInteger colorIndex = rowIndex % self.colors.count;
NSColor *color = [self.colors objectAtIndex:colorIndex];
[color set];
NSRectFill(rowFrame);
[[NSColor colorWithCalibratedWhite:0.912 alpha:1.000] set];
CGContextRef currentContext = [[NSGraphicsContext currentContext]graphicsPort];
CGContextSetLineWidth(currentContext,1.0f);
CGContextMoveToPoint(currentContext,0.0f, yRowTop - self.rowHeight);
CGContextAddLineToPoint(currentContext,NSMaxX(clipRect), yRowTop - self.rowHeight);
CGContextStrokePath(currentContext);
[[NSColor colorWithCalibratedRed:0.961 green:0.970 blue:0.985 alpha:1.000] set];
CGContextSetLineWidth(currentContext,1.0f);
CGContextMoveToPoint(currentContext,0.0f,yRowTop);
CGContextAddLineToPoint(currentContext,NSMaxX(self.bounds), yRowTop);
CGContextStrokePath(currentContext);
yStart += self.rowHeight;
rowIndex++;
}
}
当一切都说完了,我们在剪贴板视图的顶部和底部得到了漂亮的假表格视图单元行,看起来有点像这样:
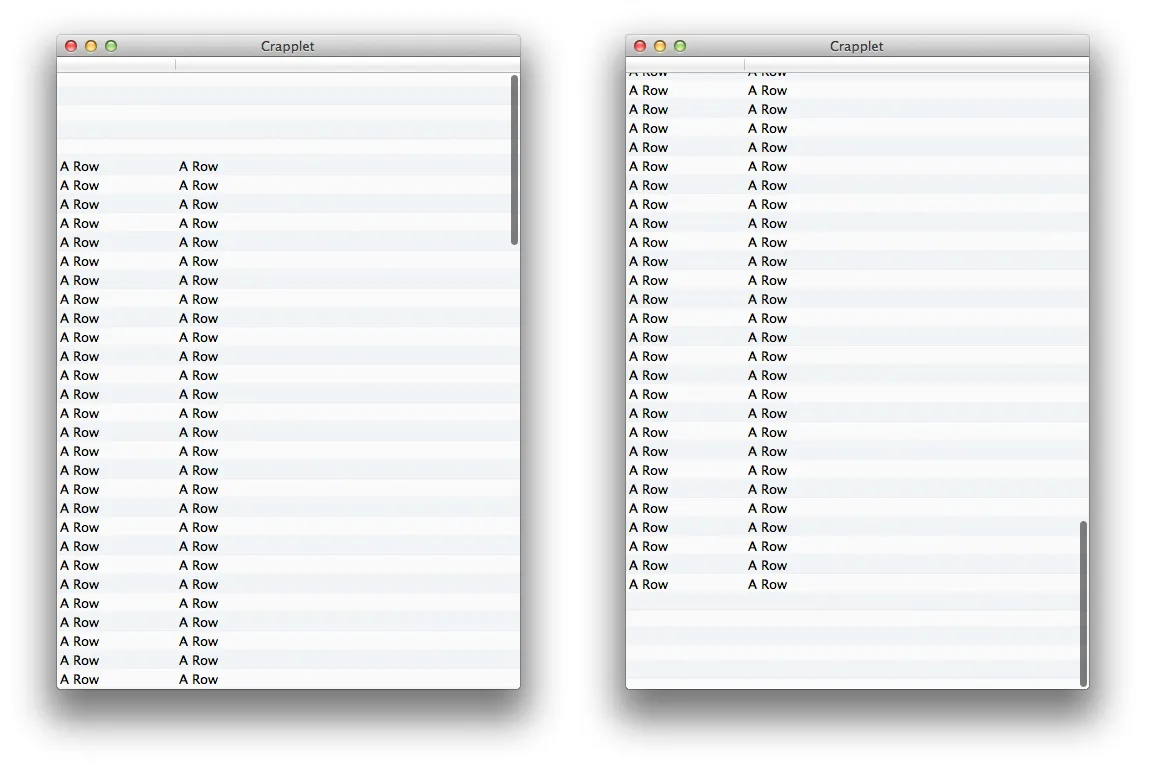
完整的子类可以在
此处找到。