我建立了一个树形结构,其中每个节点都有一个属性和一个后继节点列表。我正在尝试实现一个递归函数,从给定的节点开始遍历树(在此例中为节点“Method”),该函数将计算具有给定属性的嵌套节点的数量。最后,我希望返回单个分支中找到的最高数值。
以给定示例为例,我想找到具有属性“Loop”的嵌套节点的最大数量,该数量为3(相应的分支已用橙色标记)。
示例:
目前,我的方法如下:
这种方法的问题在于它会计算给定树中的所有循环,而不仅仅是返回嵌套分支的最大数。因此,对于给定示例,它返回7而不是我想要的3,这等于整个树中"Loops"的总数。
显然,我在递归方法方面遇到了一些问题。有人能帮帮我吗?
示例:
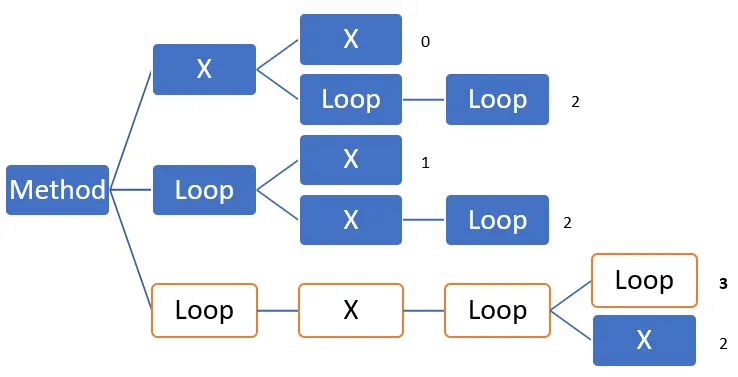
private static int getLNDforMethod(DirectedNodeInterface curNode, int currentLND) {
NodeIterator successors = curNode.getSuccessors();
while(successors.hasNext())
{
successors.next();
DirectedNodeInterface curSuc = (DirectedNodeInterface) successors.getNode();
// isLoop returns true if the given node is a loop
if(isLoop(curSuc))
{
++currentLND;
}
currentLND = getLNDforMethod(curSuc, currentLND);
}
return currentLND;
}
这种方法的问题在于它会计算给定树中的所有循环,而不仅仅是返回嵌套分支的最大数。因此,对于给定示例,它返回7而不是我想要的3,这等于整个树中"Loops"的总数。
显然,我在递归方法方面遇到了一些问题。有人能帮帮我吗?
int currentMax = 0
,并且在后继循环中看到currentMax = Math.max(currentMax,getLND...
。 - OldCurmudgeon