手势识别器
当您向视图添加手势识别器时,您可以获得一些常用的触摸事件(或手势)的通知。默认支持以下手势类型:
UITapGestureRecognizer
点按(简短地触摸屏幕一次或多次)
UILongPressGestureRecognizer
长按(长时间触摸屏幕)
UIPanGestureRecognizer
平移(在屏幕上移动手指)
UISwipeGestureRecognizer
滑动(快速移动手指)
UIPinchGestureRecognizer
捏合(将两个手指挤在一起或分开 - 通常用于缩放)
UIRotationGestureRecognizer
旋转(以圆形方向移动两个手指)
除了这些手势识别器之外,您还可以自定义自己的手势识别器。
在Interface Builder中添加手势
从对象库中拖动手势识别器到您的视图上。
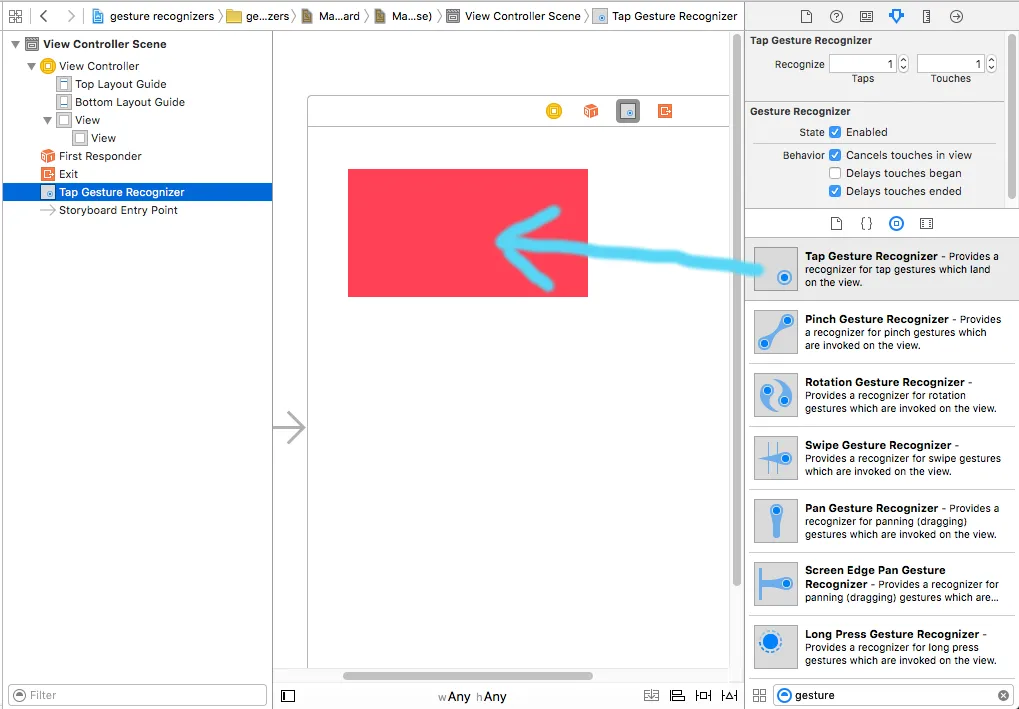
从文档大纲中控制拖动手势到您的视图控制器代码中,以创建 Outlet 和 Action。
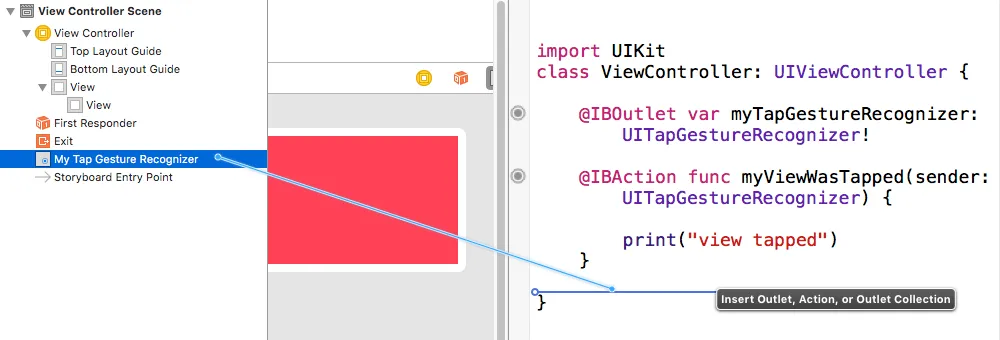
这应该是默认设置的,但也要确保您的视图中启用用户操作设置为true。
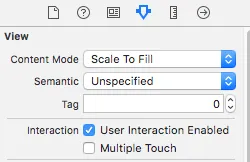
编程添加手势
要通过编程添加手势,您需要 (1) 创建一个手势识别器,(2) 将其添加到视图中,以及 (3) 创建一个在手势被识别时调用的方法。
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var myView: UIView!
override func viewDidLoad() {
super.viewDidLoad()
let tapGesture = UITapGestureRecognizer(target: self, action: #selector(handleTap(sender:)))
myView.addGestureRecognizer(tapGesture)
}
@objc func handleTap(sender: UITapGestureRecognizer) {
print("tap")
}
}
注释
sender
参数是可选的。如果您不需要对手势的引用,则可以省略它。但如果这样做,请在操作方法名称后删除 (sender:)
。
handleTap
方法的命名是任意的。使用 action: #selector(一些方法名称(sender:))
命名为您想要的任何名称。
更多示例
您可以研究我添加到这些视图中的手势识别器,以了解它们的工作原理。
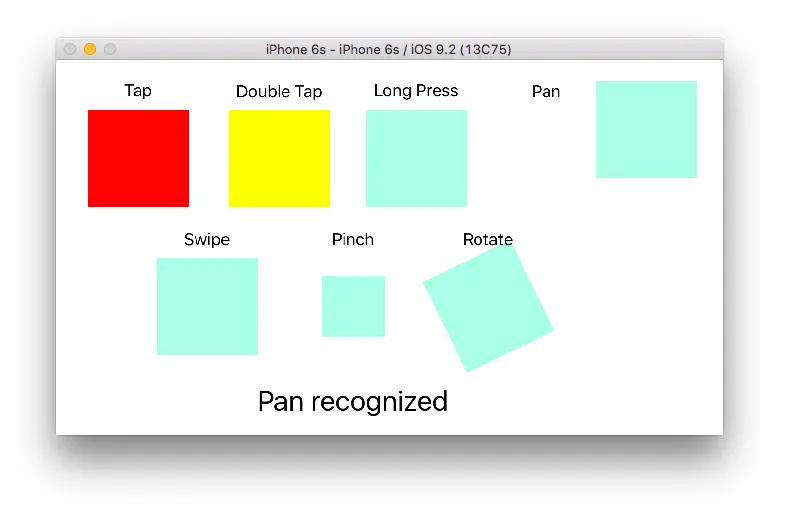
这是该项目的代码:
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var tapView: UIView!
@IBOutlet weak var doubleTapView: UIView!
@IBOutlet weak var longPressView: UIView!
@IBOutlet weak var panView: UIView!
@IBOutlet weak var swipeView: UIView!
@IBOutlet weak var pinchView: UIView!
@IBOutlet weak var rotateView: UIView!
@IBOutlet weak var label: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
let tapGesture = UITapGestureRecognizer(target: self, action: #selector(handleTap))
tapView.addGestureRecognizer(tapGesture)
let doubleTapGesture = UITapGestureRecognizer(target: self, action: #selector(handleDoubleTap))
doubleTapGesture.numberOfTapsRequired = 2
doubleTapView.addGestureRecognizer(doubleTapGesture)
let longPressGesture = UILongPressGestureRecognizer(target: self, action: #selector(handleLongPress(gesture:)))
longPressView.addGestureRecognizer(longPressGesture)
let panGesture = UIPanGestureRecognizer(target: self, action: #selector(handlePan(gesture:)))
panView.addGestureRecognizer(panGesture)
let swipeRightGesture = UISwipeGestureRecognizer(target: self, action: #selector(handleSwipe(gesture:)))
let swipeLeftGesture = UISwipeGestureRecognizer(target: self, action: #selector(handleSwipe(gesture:)))
swipeRightGesture.direction = UISwipeGestureRecognizerDirection.right
swipeLeftGesture.direction = UISwipeGestureRecognizerDirection.left
swipeView.addGestureRecognizer(swipeRightGesture)
swipeView.addGestureRecognizer(swipeLeftGesture)
let pinchGesture = UIPinchGestureRecognizer(target: self, action: #selector(handlePinch(gesture:)))
pinchView.addGestureRecognizer(pinchGesture)
let rotateGesture = UIRotationGestureRecognizer(target: self, action: #selector(handleRotate(gesture:)))
rotateView.addGestureRecognizer(rotateGesture)
}
@objc func handleTap() {
label.text = "Tap recognized"
if tapView.backgroundColor == UIColor.blue {
tapView.backgroundColor = UIColor.red
} else {
tapView.backgroundColor = UIColor.blue
}
}
@objc func handleDoubleTap() {
label.text = "Double tap recognized"
if doubleTapView.backgroundColor == UIColor.yellow {
doubleTapView.backgroundColor = UIColor.green
} else {
doubleTapView.backgroundColor = UIColor.yellow
}
}
@objc func handleLongPress(gesture: UILongPressGestureRecognizer) {
label.text = "Long press recognized"
if gesture.state == UIGestureRecognizerState.began {
let alert = UIAlertController(title: "Long Press", message: "Can I help you?", preferredStyle: UIAlertControllerStyle.alert)
alert.addAction(UIAlertAction(title: "OK", style: UIAlertActionStyle.default, handler: nil))
self.present(alert, animated: true, completion: nil)
}
}
@objc func handlePan(gesture: UIPanGestureRecognizer) {
label.text = "Pan recognized"
let location = gesture.location(in: view)
panView.center = location
}
@objc func handleSwipe(gesture: UISwipeGestureRecognizer) {
label.text = "Swipe recognized"
let originalLocation = swipeView.center
if gesture.direction == UISwipeGestureRecognizerDirection.right {
UIView.animate(withDuration: 0.5, animations: {
self.swipeView.center.x += self.view.bounds.width
}, completion: { (value: Bool) in
self.swipeView.center = originalLocation
})
} else if gesture.direction == UISwipeGestureRecognizerDirection.left {
UIView.animate(withDuration: 0.5, animations: {
self.swipeView.center.x -= self.view.bounds.width
}, completion: { (value: Bool) in
self.swipeView.center = originalLocation
})
}
}
@objc func handlePinch(gesture: UIPinchGestureRecognizer) {
label.text = "Pinch recognized"
if gesture.state == UIGestureRecognizerState.changed {
let transform = CGAffineTransform(scaleX: gesture.scale, y: gesture.scale)
pinchView.transform = transform
}
}
@objc func handleRotate(gesture: UIRotationGestureRecognizer) {
label.text = "Rotate recognized"
if gesture.state == UIGestureRecognizerState.changed {
let transform = CGAffineTransform(rotationAngle: gesture.rotation)
rotateView.transform = transform
}
}
}
注意事项
- 您可以将多个手势识别器添加到单个视图中。但为了简单起见,我并没有这样做(除了滑动手势)。如果您的项目需要,请阅读手势识别器文档。它相当易懂且有帮助。
- 上述示例存在已知问题:(1)平移视图在下一次手势事件时重置其框架。(2)第一次滑动时,滑动视图从错误的方向出现。(不过这些示例中的错误不应影响您对手势识别器工作原理的理解。)